Vue01-基础语法-模板语法-基本指令-v-bind
本文于
604
天之前发表,文中内容可能已经过时。
Vue的引入方法
Vue动态的数据展示
- 声明式编程 面试题:声明式编程和命令式编程的区别?
原生DOM都是采用的命令式编程,操作页面的元素都要获取该元素的DOM。然而在Vue中不需要操作原生的DOM,直接在data中声明数据即可。
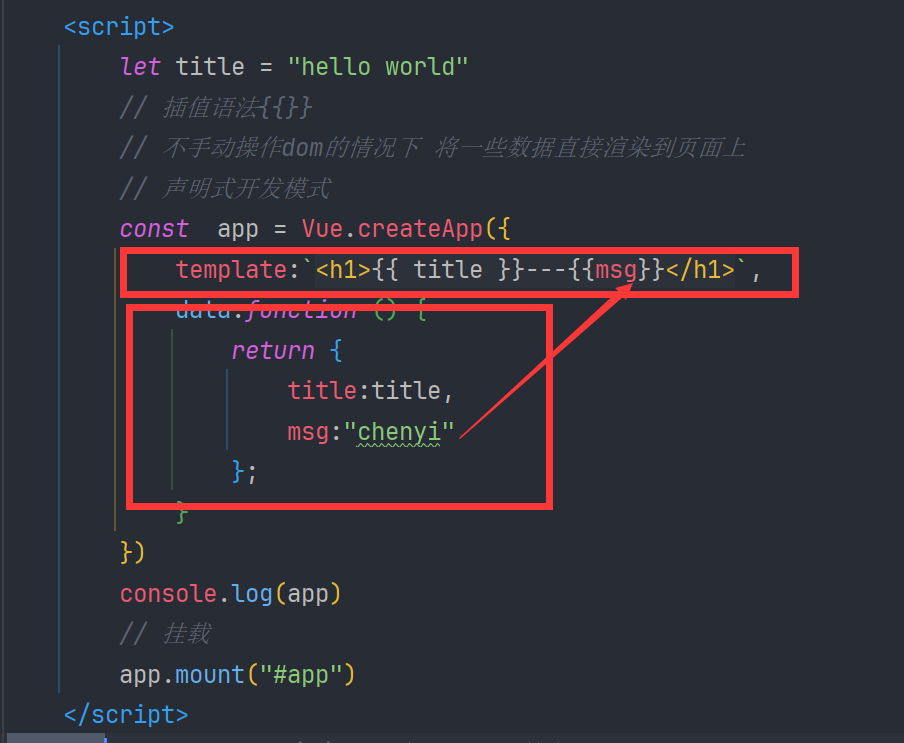
Vue展示列表数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <script> let title = "hello world" const app = Vue.createApp({ template:` <h1>{{ name }}</h1> <ul> <li v-for="(item,index) in name">{{item}}</li> </ul> `, data:function () { return { title:title, name: ["张三", "李四", "王五"], }; } }) console.log(app) app.mount("#app") </script>
|
Vue实现计数器【经典案例】
Option-api
- data 面试题:为什么data是一个函数?
- methods
Mystache双括号语法【大胡子语法】
Vue的指令
- v-once
- v-text
- v-html
- v-pre
- v-cloak
- v-memo
v-bind指令
v-bind绑定基本属性
需要动态的改变页面的属性,通过v-bind来动态的绑定
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>Title</title> <script src="./lib/Vue.js"></script> <style> </style> </head> <body> <h2>你好</h2>
<div id="app"> <div> <h1>{{name}}</h1> <img :src="url" alt="">
<a :href="href">百度一下</a> </div> <button @click="update">改变</button> </div> <script> const app = Vue.createApp({ data() { return { name:"chenyi", url:"https://www.neu.edu.cn/images/dangdaihuinew.jpg", href:"https://www.neu.edu.cn/", url2:"https://www.neu.edu.cn/images/2696de7a-6d24-4272-a7b2-7466a35d17d4.jpg", } }, methods:{ update:function (){ this.href="https://cysir.icu"; this.url = this.url === this.url2 ? this.url : this.url2 } } }); console.log(app); app.mount('#app'); </script> </body> </html>
|
v-bind绑定class属性
需要动态的改变页面的样式,通过v-bind来动态的绑定样式。【不同的写法】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>Title</title> <script src="./lib/Vue.js"></script> <style> .active{ color:red; } .demo{ font-size: 30px; } </style> </head> <body>
<div id="app"> <h1 >hello world</h1>
<button class="demo" :class="getDynamicClass()" @click="btnClick" >按钮</button>
<h1 :class="['abc','def']">hello</h1> <h1 :class="['abc',className,getDynamicClass()]">hello</h1>
</div> <script> const app = Vue.createApp({ data() { return { isActive:false, className:'why' } }, methods:{ btnClick:function (){ this.isActive = !this.isActive }, getDynamicClass:function (){ return {active:this.isActive,why:true} } } });
app.mount('#app'); </script> </body> </html>
|
v-bind绑定style属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>Title</title> <script src="./lib/Vue.js"></script> <style> .active{ color:red; } .demo{ font-size: 30px; font-family: "Agency FB"; } </style> </head> <body>
<div id="app">
<h1 :style="{color:fontColor,fontSize:fontSize}">hello world</h1>
<h2 :style="objStyle">chenyi</h2>
<h2 :style="[objStyle,{fontFamily:'Agency FB'}]">呵呵哈哈哈</h2> </div> <script> const app = Vue.createApp({ data() { return { fontColor:"green", fontSize:'60px', objStyle:{ color:'green', fontSize: '100px' } } }, methods:{ btnClick:function (){ this.isActive = !this.isActive }, getDynamicClass:function (){ return {active:this.isActive,why:true} } } });
app.mount('#app'); </script> </body> </html>
|
v-bind绑定属性名
v-bind直接绑定对象
一般在组件传值的时候可以用